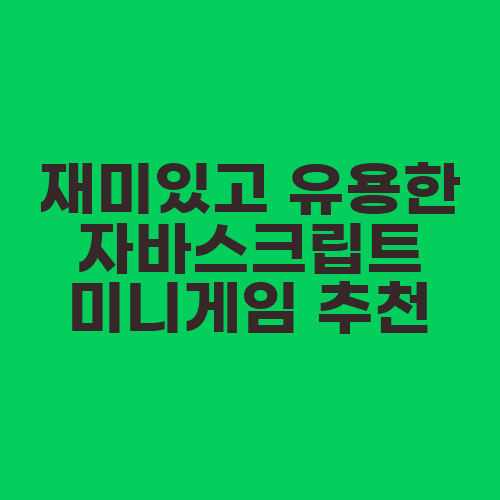
재미있고 유용한 자바스크립트 미니게임 추천
재미있고 유용한 자바스크립트 미니게임 추천
안녕하세요! 오늘은 자바스크립트로 만들어진 미니게임 중에서 재미있고 유용한 몇 가지를 추천해 드릴게요. 이 게임들은 모두 간단한 규칙과 룰로 이루어져 있어 누구나 쉽게 즐길 수 있습니다. 함께 살펴보시죠!
1. 야구 게임
function baseballGame() {
const answer = [];
for (let i = 0; i < 3; i++) {
let random = Math.floor(Math.random() * 10);
while (answer.includes(random)) {
random = Math.floor(Math.random() * 10);
}
answer.push(random);
}
let strike = 0;
let ball = 0;
let tryCount = 0;
while (strike < 3) {
const playerInput = prompt('0~9 사이의 숫자 세 개를 입력하세요. (각 숫자는 띄어쓰기로 구분)');
const playerArray = playerInput.split(' ').map((num) => parseInt(num));
strike = 0;
ball = 0;
for (let i = 0; i < answer.length; i++) {
if (answer[i] === playerArray[i]) {
strike++;
} else if (answer.includes(playerArray[i])) {
ball++;
}
}
tryCount++;
alert(`현재 ${tryCount}번째 시도! 결과: ${strike} 스트라이크, ${ball} 볼`);
}
alert(`숫자 맞추기 성공! ${tryCount}번 만에 성공했습니다!`);
}
자바스크립트로 만든 가장 대표적인 미니게임 중 하나인 야구 게임입니다. 이 게임은 간단한 숫자 맞추기 게임으로, 0부터 9까지의 숫자 중 서로 다른 3개를 뽑아 놓은 후, 플레이어는 이 숫자를 맞추는 게임입니다. 숫자와 위치가 맞으면 스트라이크, 숫자는 맞지만 위치가 틀리면 볼이 됩니다. 총 3개의 스트라이크를 얻어야 게임에서 이길 수 있습니다.
2. 스네이크 게임
const canvas = document.getElementById('game');
const ctx = canvas.getContext('2d');
const width = canvas.width;
const height = canvas.height;
class Snake {
constructor() {
this.body = [
{ x: 3, y: 2 },
{ x: 2, y: 2 },
{ x: 1, y: 2 },
{ x: 0, y: 2 },
];
this.direction = 'right';
}
move() {
const head = this.body[0];
if (this.direction === 'right') {
this.body.unshift({ x: head.x + 1, y: head.y });
} else if (this.direction === 'left') {
this.body.unshift({ x: head.x - 1, y: head.y });
} else if (this.direction === 'up') {
this.body.unshift({ x: head.x, y: head.y - 1 });
} else if (this.direction === 'down') {
this.body.unshift({ x: head.x, y: head.y + 1 });
}
this.body.pop();
}
draw() {
ctx.clearRect(0, 0, width, height);
this.body.forEach((cell) => {
ctx.fillRect(cell.x * 10, cell.y * 10, 10, 10);
});
}
changeDirection(direction) {
if (
(this.direction === 'up' && direction === 'down') ||
(this.direction === 'down' && direction === 'up') ||
(this.direction === 'left' && direction === 'right') ||
(this.direction === 'right' && direction === 'left')
) {
return;
} else {
this.direction = direction;
}
}
}
class Food {
constructor() {
this.position = { x: 0, y: 0 };
}
place() {
const x = Math.floor(Math.random() * (width / 10));
const y = Math.floor(Math.random() * (height / 10));
this.position.x = x;
this.position.y = y;
}
draw() {
ctx.fillRect(this.position.x * 10, this.position.y * 10, 10, 10);
}
}
const snake = new Snake();
const food = new Food();
food.place();
document.addEventListener('keydown', (e) => {
if (e.key === 'ArrowUp') {
snake.changeDirection('up');
} else if (e.key === 'ArrowDown') {
snake.changeDirection('down');
} else if (e.key === 'ArrowLeft') {
snake.changeDirection('left');
} else if (e.key === 'ArrowRight') {
snake.changeDirection('right');
}
});
setInterval(() => {
snake.move();
if (snake.body[0].x === food.position.x && snake.body[0].y === food.position.y) {
snake.body.push({});
food.place();
}
snake.draw();
food.draw();
}, 100);
스네이크 게임은 오래전부터 여러 게임 기기에서 즐겨지던 대표적인 게임입니다. 이 게임은 뱀이 먹이를 먹으면서 길어지는 모습을 그리는 게임으로, 자바스크립트로 만든 버전은 캔버스를 이용해 구현됩니다. 방향키를 통해 뱀의 이동 방향을 조작하고 먹이를 먹으며 게임을 진행합니다.
3. 타일 게임
const gameContainer = document.getElementById('game-container');
const NUM_TILES = 25;
const colors = ['red', 'blue', 'green', 'yellow'];
function randomTileColor() {
const randomIndex = Math.floor(Math.random() * colors.length);
return colors[randomIndex];
}
function renderTiles() {
for (let i = 0; i < NUM_TILES; i++) {
const tile = document.createElement('div');
tile.classList.add('tile');
tile.style.backgroundColor = randomTileColor();
gameContainer.appendChild(tile);
tile.addEventListener('click', () => {
if (selectedTile) {
const selectedColor = selectedTile.style.backgroundColor;
const clickedColor = tile.style.backgroundColor;
if (selectedColor === clickedColor) {
gameContainer.removeChild(selectedTile);
gameContainer.removeChild(tile);
selectedTile = null;
} else {
selectedTile.classList.remove('selected');
selectedTile = null;
}
} else {
selectedTile = tile;
tile.classList.add('selected');
}
});
}
}
let selectedTile = null;
renderTiles();
타일 게임은 클릭을 통해 같은 색상의 타일을 제거하는 게임입니다. 이 게임을 자바스크립트로 만든 버전은 간단한 HTML과 CSS로 타일을 그린 뒤, 자바스크립트를 이용해 색상을 무작위로 지정하여 게임을 시작합니다. 같은 색상의 타일을 클릭하면 타일이 제거되며, 게임이 끝날 때까지 이를 반복합니다.
이렇게 세 가지의 재미있고 유용한 자바스크립트 미니게임을 소개해 드렸습니다. 이 게임들은 모두 간단한 코드와 규칙으로 이루어져 있어, 자바스크립트 초보자도 충분히 만들어 볼 수 있습니다. 새로운 자바스크립트 프로젝트를 할 때, 이런 미니게임들을 만들어 프로그래밍 실력을 향상시켜보세요!
자바스크립트 미니게임, 자바스크립트 초보자, 야구 게임, 스네이크 게임, 타일 게임