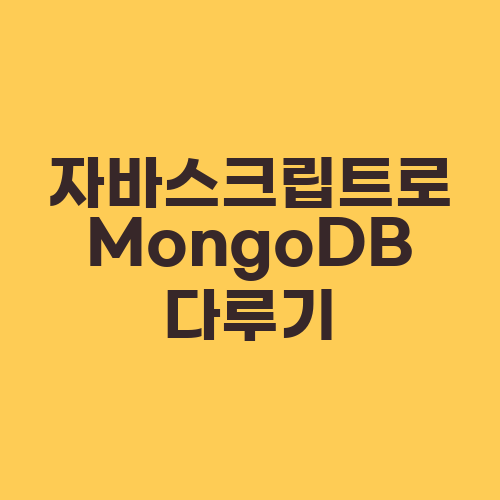
자바스크립트로 MongoDB 다루기
자바스크립트로 MongoDB 다루기
MongoDB 연결하기
const MongoClient = require('mongodb').MongoClient;
const uri = "mongodb+srv://:@cluster0.mongodb.net/test?retryWrites=true&w=majority";
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
client.connect(err => {
const collection = client.db("test").collection("devices");
// perform actions on the collection object
client.close();
});
작성한 코드는 MongoDB에 연결하기 위한 기본적인 방법입니다. `
데이터 추가하기
const insertDocuments = function(db, callback) {
// Get the documents collection
const collection = db.collection('documents');
// Insert some documents
collection.insertMany([
{a : 1}, {a : 2}, {a : 3}
], function(err, result) {
console.log("Inserted 3 documents into the collection");
callback(result);
});
}
위 코드는 `documents` 컬렉션에 3개의 도큐먼트를 추가하는 코드입니다. `insertMany()` 함수를 이용하여 한번에 여러 개의 도큐먼트를 추가할 수 있습니다. 추가가 완료되면 `callback()` 함수를 호출하여 결과를 처리할 수 있습니다.
데이터 조회하기
const findDocuments = function(db, callback) {
// Get the documents collection
const collection = db.collection('documents');
// Find some documents
collection.find({}).toArray(function(err, docs) {
console.log("Found the following records");
console.log(docs)
callback(docs);
});
}
위 코드는 `documents` 컬렉션의 모든 도큐먼트를 가져오는 코드입니다. `find()` 함수를 이용하여 쿼리를 작성하면 원하는 조건의 도큐먼트만 가져올 수 있습니다.
데이터 수정하기
const updateDocument = function(db, callback) {
// Get the documents collection
const collection = db.collection('documents');
// Update document where a is 2, set b equal to 1
collection.updateOne({ a : 2 }, { $set: { b : 1 } }, function(err, result) {
console.log("Updated the document with the field a equal to 2");
callback(result);
});
}
위 코드는 `documents` 컬렉션에서 `a` 값이 `2`인 도큐먼트의 `b` 값을 `1`로 수정하는 코드입니다. `updateOne()` 함수를 이용하여 한 개의 도큐먼트만 수정할 수 있습니다.
데이터 삭제하기
const removeDocument = function(db, callback) {
// Get the documents collection
const collection = db.collection('documents');
// Delete document where a is 3
collection.deleteOne({ a : 3 }, function(err, result) {
console.log("Removed the document with the field a equal to 3");
callback(result);
});
}
위 코드는 `documents` 컬렉션에서 `a` 값이 `3`인 도큐먼트를 삭제하는 코드입니다. `deleteOne()` 함수를 이용하여 한 개의 도큐먼트만 삭제할 수 있습니다.
자바스크립트로 MongoDB 데이터베이스를 다루는 방법을 알아보았습니다. Node.js 환경에서 MongoDB를 다룰 때 매우 유용한 기능입니다.
(키워드: 자바스크립트 MongoDB)