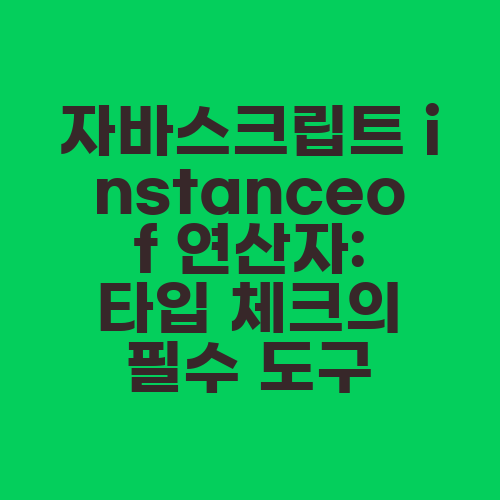
자바스크립트 instanceof 연산자: 타입 체크의 필수 도구
자바스크립트 instanceof 연산자: 타입 체크의 필수 도구
자바스크립트의 타입 체크
자바스크립트는 동적 타입 언어로, 변수의 타입이 실행 시점에 결정됩니다. 이는 유연성을 제공하고, 코드 작성을 더욱 쉽게 만들어 주지만, 런타임 오류를 발생시킬 가능성도 높입니다. 그래서 자바스크립트에서는 변수의 타입을 체크하는 여러 방법이 제공됩니다.
instanceof 연산자
그 중에서 가장 대표적인 방법이 바로 instanceof 연산자입니다. 이 연산자는 특정 객체가 지정한 클래스의 인스턴스인지 판단하는 데 사용됩니다. 예를 들어, 다음과 같이 Person 클래스가 있다고 가정해 봅시다.
class Person {
constructor(name) {
this.name = name;
}
}
이제 Person 클래스의 인스턴스를 만들고, instanceof 연산자를 이용해 체크해 보겠습니다.
const person = new Person('John');
if (person instanceof Person) {
console.log('person is an instance of Person class');
} else {
console.log('person is NOT an instance of Person class');
}
위 코드에서 instanceof 연산자는 person 객체가 Person 클래스의 인스턴스인지를 체크합니다. person은 Person 클래스의 인스턴스이므로, 위 코드는 “person is an instance of Person class”를 출력합니다.
중첩 클래스에서 instanceof 연산자 사용하기
instanceof 연산자는 중첩 클래스에서도 잘 작동합니다. 예를 들어, 다음과 같이 Person 클래스 안에 Employee 클래스를 중첩해서 만들어 봅시다.
class Person {
constructor(name) {
this.name = name;
}
class Employee {
constructor(title) {
this.title = title;
}
}
}
이제 Employee 클래스의 인스턴스를 만들고, instanceof 연산자를 이용해 체크해 보겠습니다.
const employee = new Person.Employee('Engineer');
if (employee instanceof Person.Employee) {
console.log('employee is an instance of Person.Employee class');
} else {
console.log('employee is NOT an instance of Person.Employee class');
}
위 코드에서 instanceof 연산자는 employee 객체가 Person 클래스의 Employee 클래스의 인스턴스인지를 체크합니다. employee는 Person 클래스의 Employee 클래스의 인스턴스이므로, 위 코드는 “employee is an instance of Person.Employee class”를 출력합니다.
결론
자바스크립트 개발자라면 instanceof 연산자를 반드시 숙지하고 있어야 합니다. 이 연산자를 이용하면, 변수나 객체가 원하는 타입인지 확인할 수 있습니다. 즉, 예상치 못한 런타임 오류를 방지하며 코드의 안정성을 높일 수 있게 됩니다.
자바스크립트 instanceof 연산자는 객체의 타입 체크를 위한 기본 도구입니다.