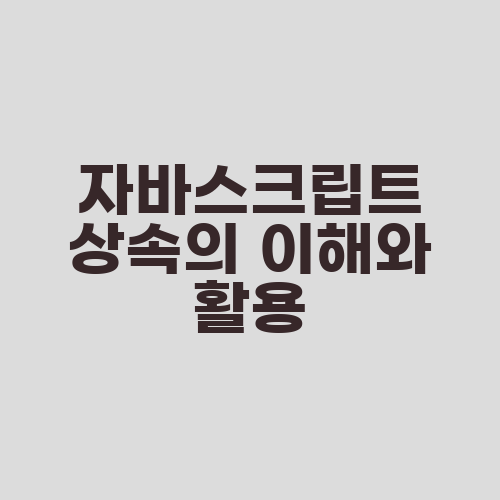
자바스크립트 상속의 이해와 활용
자바스크립트 상속의 이해와 활용
상속이란?
자바스크립트에서 상속은 부모 객체에서 자식 객체로 속성과 메소드를 전달하는 것을 의미합니다. 상속은 코드의 재사용성을 높이고 유지보수를 쉽게 만들어줍니다.
프로토타입 상속
프로토타입을 이용한 상속은 자바스크립트에서 기본적으로 사용되는 상속 방법입니다. 프로토타입을 상속받은 객체는 부모 객체의 메소드와 속성을 사용할 수 있습니다.
function Parent() {
this.name = 'Parent';
}
Parent.prototype.sayHello = function() {
console.log('Hello from ' + this.name);
}
function Child() {
}
Child.prototype = new Parent();
Child.prototype.constructor = Child;
const child = new Child();
child.sayHello(); // "Hello from Child"
클래스 상속
ES6에서는 클래스를 이용한 상속 방법도 제공됩니다. 클래스 상속은 기존의 객체 지향 언어에서 사용하는 상속과 유사합니다.
class Parent {
constructor() {
this.name = 'Parent';
}
sayHello() {
console.log('Hello from ' + this.name);
}
}
class Child extends Parent {
constructor() {
super();
}
}
const child = new Child();
child.sayHello(); // "Hello from Child"
결론
자바스크립트에서는 프로토타입 상속과 클래스 상속 두 가지 방법을 이용하여 상속을 구현할 수 있습니다. 이러한 상속 방법을 이용하면 코드의 재사용성을 높이고 유지보수를 쉽게 만들 수 있습니다.
**자바스크립트 상속**은 부모 객체에서 자식 객체로 속성과 메소드를 전달하는 것을 의미합니다. 프로토타입 상속과 클래스 상속 두 가지 방법을 이용하여 구현할 수 있습니다.