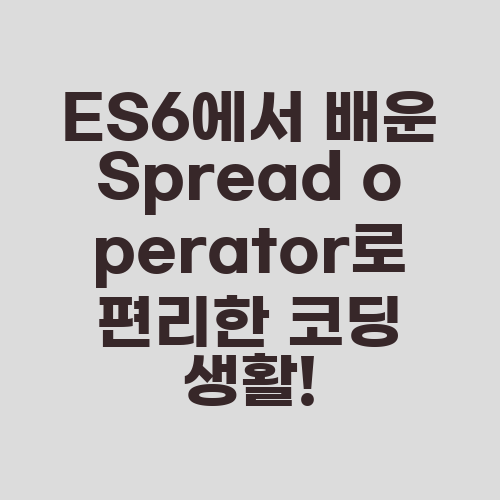
ES6에서 배운 Spread operator로 편리한 코딩 생활!
ES6에서 배운 Spread operator로 편리한 코딩 생활!
ES6에서 도입된 Spread operator는 자바스크립트 개발자들에게 큰 도움이 됩니다. 간결한 코드로 변수를 복사, 배열과 객체를 합치고, 함수 인자를 전달할 수 있습니다.
변수 복사하기
const oldArray = [1, 2, 3];
const newArray = [...oldArray];
console.log(newArray); // [1, 2, 3]
Spread operator를 사용하면 변수를 간단하게 복사할 수 있습니다. 예제에서는 기존 배열을 새 배열에 할당합니다.
배열 합치기
const fruits = ['apple', 'banana'];
const vegetables = ['carrot', 'tomato'];
const produce = [...fruits, ...vegetables];
console.log(produce); // ['apple', 'banana', 'carrot', 'tomato']
Spread operator를 사용하면 배열을 병합할 수 있습니다. 배열 각각의 요소를 하나씩 전개하여 새로운 배열을 만듭니다.
객체 합치기
const user = { name: 'John', age: 30 };
const details = { email: 'john@example.com', phone: '123-456-7890' };
const merged = { ...user, ...details };
console.log(merged); // { name: 'John', age: 30, email: 'john@example.com', phone: '123-456-7890' }
Spread operator를 사용하면 객체를 병합할 수 있습니다. 객체 각각의 프로퍼티를 하나씩 전개하여 새로운 객체를 만듭니다.
함수 인자 전달하기
const numbers = [1, 2, 3];
const sum = (a, b, c) => a + b + c;
const total = sum(...numbers);
console.log(total); // 6
Spread operator를 사용하면 배열을 함수의 인자로 전달할 수 있습니다.
자바스크립트 Spread operator는 간단하면서도 매우 편리한 기능입니다. 새로운 개발 프로젝트를 시작하거나 기존 코드를 업데이트하는 경우 Spread operator를 활용하면 코드의 가독성과 효율성을 높일 수 있습니다.
자바스크립트, Spread operator