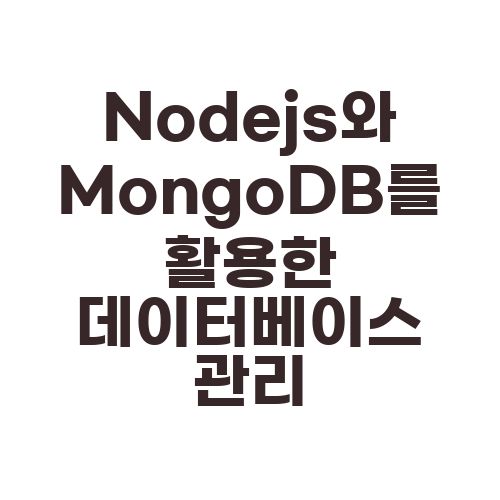
Nodejs와 MongoDB를 활용한 데이터베이스 관리
Nodejs와 MongoDB를 활용한 데이터베이스 관리
MongoDB란 무엇인가?
MongoDB는 NoSQL의 대표적인 데이터베이스이며, JSON 형태의 도큐먼트를 저장하고 검색할 수 있는 데이터베이스입니다. 이러한 특징 때문에 확장성과 유연성이 높아서 최근에는 많은 기업에서 사용하고 있습니다.
Nodejs와 MongoDB를 연동하기
Node.js는 MongoDB를 관리하고 처리할 수 있는 첫 번째 클래스 도구입니다. Node.js에서 MongoDB를 사용하려면 모듈을 설치해야합니다. 모듈은 NPM(Node Package Manager)를 통해 설치할 수 있습니다. 다음은 MongoDB Node.js 드라이버를 설치하기 위한 명령어입니다.
npm install mongodb
Nodejs에서의 CRUD 작업
MongoDB Node.js 드라이버로 Node.js에서 CRUD 작업을 수행할 수 있습니다. 이 부분에서는 Node.js에서 MongoDB를 사용하여 CRUD(Create, Read, Update, Delete) 작업을 수행하는 방법을 살펴보겠습니다.
Create
MongoDB에 데이터를 추가하려면 insertOne() 또는 insertMany() 메서드를 사용할 수 있습니다.
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'myproject';
const client = new MongoClient(url);
client.connect((err) => {
console.log("Connected successfully to server");
const db = client.db(dbName);
db.collection('documents').insertOne({
name: "Jane",
age: 24
}, (err, result) => {
console.log("Inserted 1 document into the collection");
});
client.close();
});
Read
MongoDB에서 데이터를 가져오려면 find() 메서드를 사용해야 합니다.
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'myproject';
const client = new MongoClient(url);
client.connect((err) => {
console.log("Connected successfully to server");
const db = client.db(dbName);
db.collection('documents').find({}).toArray((err, docs) => {
console.log("Found the following records");
console.log(docs);
});
client.close();
});
Update
MongoDB 데이터를 업데이트하려면 updateOne() 또는 updateMany() 메서드를 사용할 수 있습니다.
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'myproject';
const client = new MongoClient(url);
client.connect((err) => {
console.log("Connected successfully to server");
const db = client.db(dbName);
db.collection('documents').updateOne(
{ name: "Jane" },
{ $set: { age: 25 } },
(err, result) => {
console.log("Updated the document with the field 'age:25'");
}
);
client.close();
});
Delete
MongoDB에서 데이터를 삭제하려면 deleteOne() 또는 deleteMany() 메서드를 사용합니다.
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'myproject';
const client = new MongoClient(url);
client.connect((err) => {
console.log("Connected successfully to server");
const db = client.db(dbName);
db.collection('documents').deleteOne({ name: "Jane" }, (err, result) => {
console.log("Removed the document with the field 'name:Jane'");
});
client.close();
});
결론
Node.js에서 MongoDB를 사용하는 방법과 CRUD 작업을 수행하는 방법에 대해 살펴보았습니다. 둘을 함께 사용하여 MongoDB를 더욱 유연하고 확장성있게 사용할 수 있습니다.
자바스크립트, 노드, MongoDB, 데이터베이스
이 글은 Nodejs와 MongoDB를 활용한 데이터베이스 관리에 대한 가장 기본적인 개념을 다루었습니다. Node.js를 사용하면 데이터베이스를 빠르고 쉽게 관리할 수 있으며, MongoDB를 함께 사용하면 유연하고 확장 가능한 애플리케이션을 빌드할 수 있습니다. 이러한 이점들 때문에 최근에는 Node.js와 MongoDB를 함께 사용하는 기업들이 늘어나고 있습니다.